Free Gift Products in Shopping Cart
Published on April 30th, 2024
Adding Free Gift Products to the cart automatically
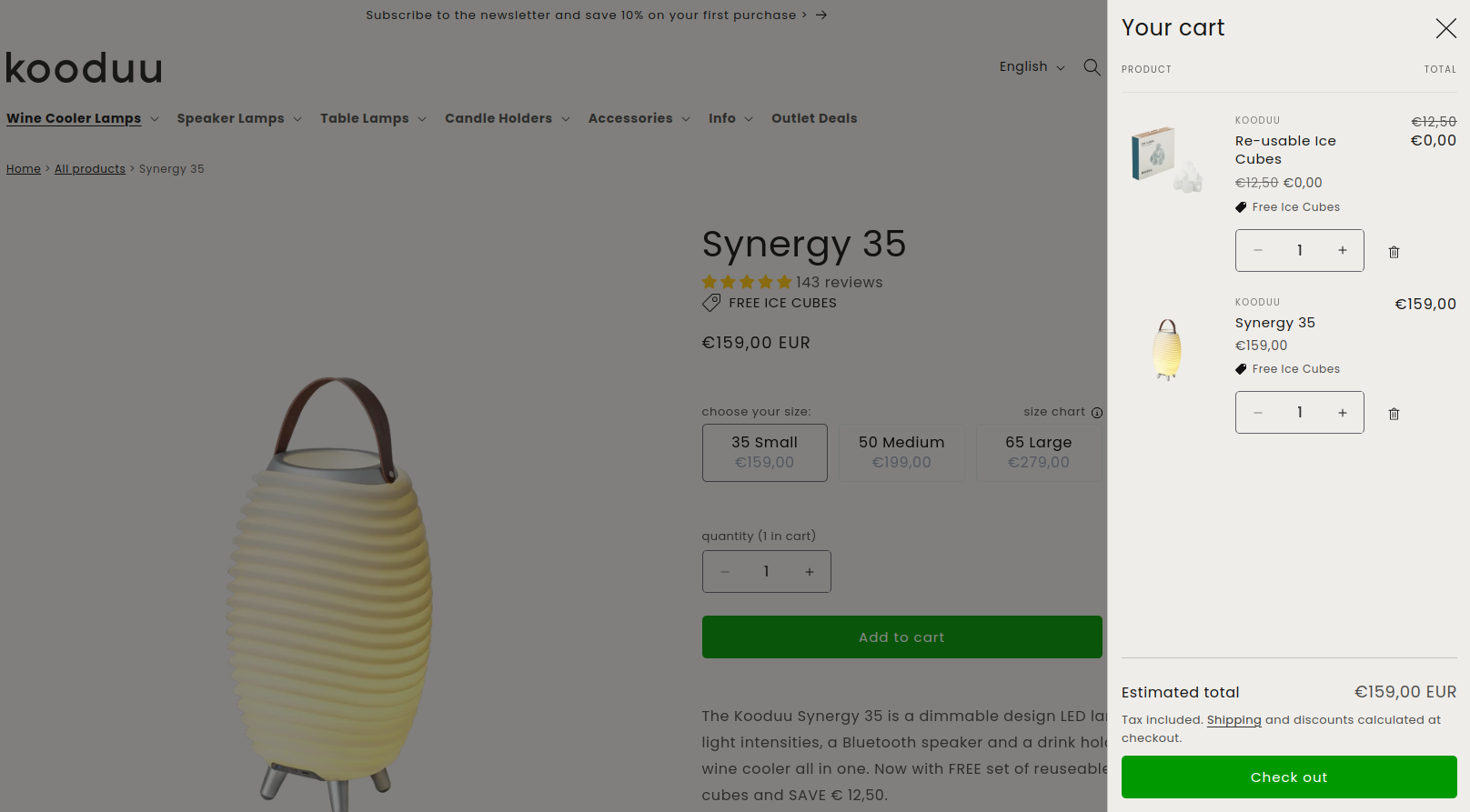
Shopify by default does not have an option to automatically add a free gift product to the cart. There is however a way to make this work.
To start, create a new file snippets/free-product.liquid
and add the following code:
{% assign freegift_product1 = all_products['free-gift-1'].variants.first.id %} {% assign freegift_product2 = all_products['free-gift-2'].variants.first.id %} <script type="text/javascript"> window.freeProductEnabled = true; window.freeProducts = function(product, quantity) { addToCart(product, quantity); } window.productLinesWithFreeGift = { 'Product Type 1': [{{ freegift_product1 }}], 'Product Type 2': [{{ freegift_product2 }}], }; function addToCart(product_id, quantity) { const config = fetchConfig('javascript'); config.headers['X-Requested-With'] = 'XMLHttpRequest'; delete config.headers['Content-Type']; const formData = new FormData(); formData.append('quantity', quantity); formData.append('form_type', 'product'); formData.append('id', product_id); const cart = document.querySelector('cart-drawer'); if (cart) { formData.append('sections', cart.getSectionsToRender().map((section) => section.id)); formData.append('sections_url', window.location.pathname); cart.setActiveElement(document.activeElement); } config.body = formData; fetch(`${routes.cart_add_url}`, config) .then((response) => response.json()) .then((response) => { cart.renderContents(response); }) .catch((e) => { console.error(e); }) .finally(() => { if (cart && cart.classList.contains('is-empty')) cart.classList.remove('is-empty'); cart.querySelector('.loading-overlay__spinner').classList.add('hidden'); }); } </script>
Replace the free gift products (line 1 and 2) with the ones you want to give to your customer. Then also replace the product types (line 9 and 10) with the products you want the free gifts to apply to. You can enter the names, or a part of the name of those products.
Then we need to add a piece of code to the bottom of the following code in assets/product-form.js
:
} else { this.cart.renderContents(response); }
You need to add the following code:
if(window.freeProductEnabled) { const quantity = document.querySelector('product-info .quantity__input') ? document.querySelector('product-info .quantity__input').value : 1; for(const productName in window.productLinesWithFreeGift) { const products = window.productLinesWithFreeGift[productName]; for(let i = 0; i < products.length; i++) { const product = products[i]; if(response.title.includes(productName)) { setTimeout(function(){ window.freeProducts(product, quantity); }, i*250); // Add a delay. If we add cart items too fast, only the last item will be added } } } }
Then finally add the discount rules that give a 100% discount on the free gift products.